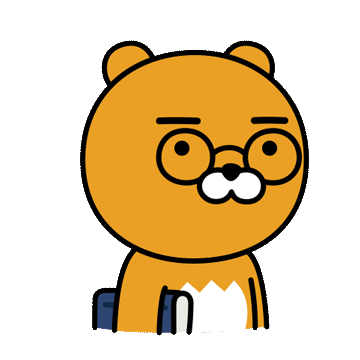
Dictionary란?
- Dictionary는 키(key)와 값(value)을 쌍으로 저장하는 클래스입니다. 이는 해시 테이블을 기반으로 하여 빠른 데이터 검색 및 조회를 할 수 있습니다.
- Dictionary는 System.Collections.Generic 에 속해 있습니다.
- 키의 유일성: 각 키는 유일해야 하며, 중복된 키를 허용하지 않습니다.
- 값의 중복 허용: 값은 중복될 수 있습니다. 즉, 여러 개의 다른 키가 동일한 값을 가질 수 있습니다.
<출처:https://www.learnerslesson.com/CSHARP/Images/C-Sharp-Dictionary1.png >
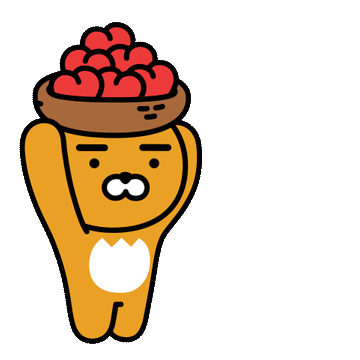
Dictionary를 사용해보자!
1. Dictionary 선언 및 초기화
//키는 문자열, 밸류는 정수형을 가진 딕셔너리
Dictionary<string, int> dic = new Dictionary<string, int>();
2. Add(key, value) - 데이터 추가
Dictionary<string, int> dic = new Dictionary<string, int>();
dic.Add("짱구", 5);
dic.Add("봉미선", 29);
dic["신형만"] = 30; //다른 방법으로 데이터 추가.
foreach (var item in dic)
{
Debug.Log(item.Key +" = " + item.Value);
}
3. Remove(key, value) - 지정된 키와 연결된 값 제거
- Remove를 사용하게 되면 지정된 키와 연결된 값이 전부 제거됩니다.
Dictionary<string, int> dic = new Dictionary<string, int>();
dic.Add("짱구", 5);
dic.Add("봉미선", 29);
dic["신형만"] = 30;
dic.Remove("봉미선");
foreach (var item in dic)
{
Debug.Log(item.Key +" = " + item.Value);
}
- 키 값은 남겨두고 연결된 밸류 값만 제거하고 싶으면 default(값의 데이터 형식)를 사용하면 됩니다. 이 때 키는 데이터가 남아있고 연결된 값은 사라지며, 출력하면 0이 나옵니다.
Dictionary<string, int> dic = new Dictionary<string, int>();
dic.Add("짱구", 5);
dic.Add("봉미선", 29);
dic["신형만"] = 30;
dic["봉미선"] = default(int);
foreach (var item in dic)
{
Debug.Log(item.Key +" = " + item.Value);
}
4. ContainsKey(key)와 ContainsValue(value) - 지정된 키/밸류가 해당 dictionary에 존재하는지 확인
Dictionary<string, int> dic = new Dictionary<string, int>();
dic.Add("짱구", 5);
dic.Add("봉미선", 29);
dic["신형만"] = 30;
//"짱구"가 dic의 키에 있는지 확인.
bool findKey = dic.ContainsKey("짱구");
//100이 dic의 밸류에 있는지 확인.
bool findValue = dic.ContainsValue(100);
Debug.Log(findKey);
Debug.Log(findValue);
5. Keys/Values - 모든 키/밸류를 나타내는 컬렉션을 가져옵니다.
Dictionary<string, int> dic = new Dictionary<string, int>();
dic.Add("짱구", 5);
dic.Add("봉미선", 29);
dic["신형만"] = 30;
var keys = dic.Keys; //dic의 모든 키를 전달.
var values = dic.Values; //dic의 모든 밸류를 전달.
foreach (var key in keys)
{
Debug.Log(key);
}
Debug.Log("----------------------");
foreach (var value in values)
{
Debug.Log(value);
}
'C# > 자료구조 이해하기' 카테고리의 다른 글
C# 오름차순과 내림차순 정렬에 대해 알아보자! (0) | 2024.03.10 |
---|---|
C# List(리스트)에 대해 알아보자! (0) | 2024.03.10 |
C# 스택(Stack)에 대해 알아보자! (0) | 2024.03.05 |
C# 큐(Queue)에 대해 알아보자! (0) | 2024.03.05 |
C# 배열(Array)을 알아보자! (2) | 2024.03.05 |